Adding New Modules
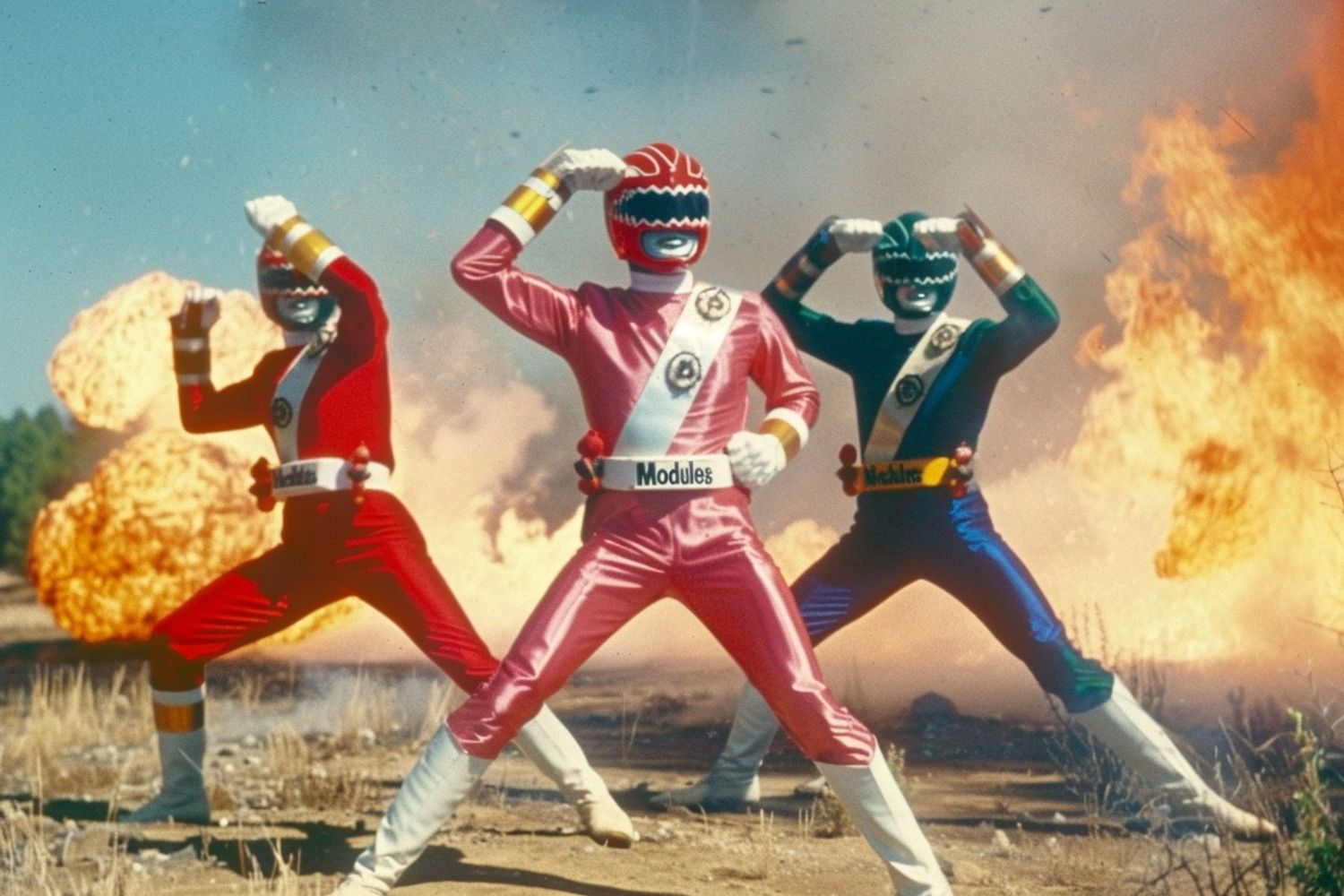
Adding new custom modules is as easy as 3 steps:
- Define the schema.
- Create a frontend component.
- Assign the component in
<Modules/>
.
Define the schema in Sanity๐
Create a new object
schema type in the modules directory:
import { defineField, defineType } from 'sanity'
export default defineType({
name: 'custom-module',
title: 'Custom module',
type: 'object',
fields: [
// ...
],
})
Import the newly created schema file:
// documents
// objects
// modules
import customModule from './modules/custom-module'
export const schemaTypes = [
// documents
// objects
// modules
customModule,
]
Then, include the module name to all page documents where you'd like to use the module:
export default defineType({
name: 'page',
title: 'Page',
type: 'document',
fields: [
// ...
defineField({
name: 'modules',
type: 'array',
of: [
// ...
{ type: 'custom-module' },
],
}),
],
})
Create the Frontend Component๐
Create the React component in the Next.js frontend. I recommend defining the schema as TypeScript types within this component file:
export default function CustomModule({}: Props) {
return (
<section className="section">
...
</section>
)
}
Then, add a new case in the <Modules/>
handler component:
import CustomModule from './CustomModule'
export default function Modules({ modules }: { modules?: Sanity.Module[] }) {
return (
<>
{modules?.map((module) => {
switch (module._type) {
// ...
case 'custom-module':
return <CustomModule {...module} key={module._key} />
default:
return <div data-type={module._type} key={module._key} />
}
})}
</>
)
}
Joining References with GROQ๐
If your new modules includes references to other Sanity documents, you'll need to update the module GROQ query to "join" the references.
export const modulesQuery = groq`
...,
_type == 'custom-module' => {
yourReferencedItems[]->
},
`
Now you'll have access to the referenced documents' fields.
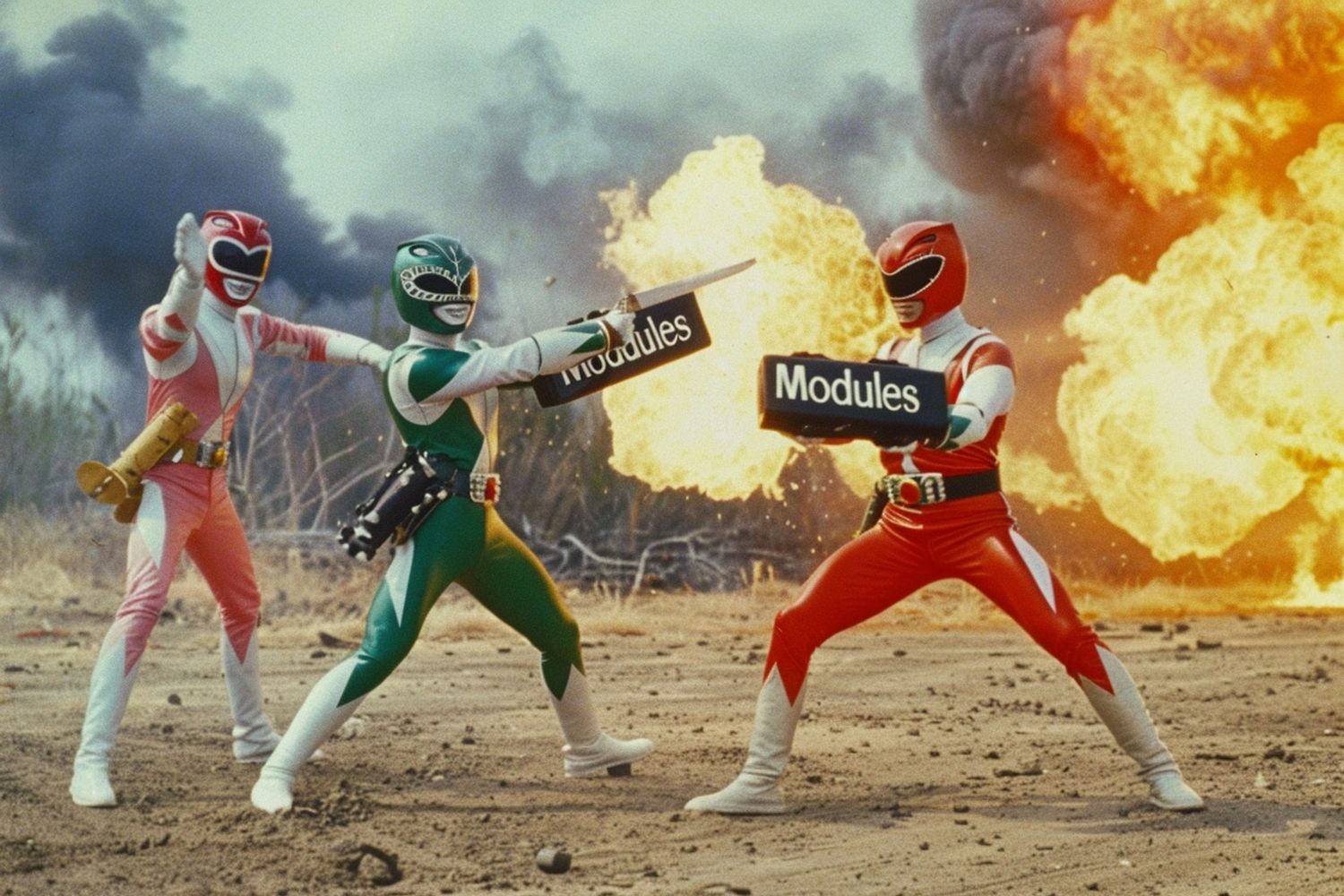